- Published on
Software Architecture: Conventions and Principles
- Authors
In Part 1 of the Software Architecture Series, we introduced Architecture Paradigms, where we explored monolithic, microservices, and event-driven architectures. This second part dives into the conventions and principles guiding the development of both frontend and backend systems.
As a reminder, here are the four parts of the series:
- Part 1: Architecture Paradigms: Exploring common architecture paradigms such as monolithic, microservices, and event-driven architectures.
- Part 2: Architecture Conventions and Principles: Deep dive into best practices, principles like SOLID, and architectural patterns such as CQRS, Repository Pattern, etc.
- Part 3: Solution Architecture: How to design a solution that meets business needs and scales with your organization.
- Part 4: Enterprise Architecture: Looking at the broader perspective of system integrations, scalability, and the role of enterprise architecture in large organizations.
Understanding the fundamental conventions and principles helps design robust, scalable, and secure systems that work efficiently in various sectors.
Frontend Architecture Conventions and Principles
Frontend architecture defines how user interfaces (UIs) are structured, the flow of data, and the methods to enhance user experience. Here are key conventions and principles:
Server-Side Rendering (SSR) vs. Client-Side Rendering (CSR)
SSR involves rendering the initial HTML of a webpage on the server side, improving SEO and load times for initial views. CSR, common in Single Page Applications (SPA), renders components on the client side, enhancing interactivity but sometimes leading to slower initial loads.
Comparison of SSR and CSR
Principles:
- Choose SSR for SEO-intensive pages (like eCommerce landing pages).
- Use CSR when high interactivity (e.g., dashboards) is a priority.
Serverless and Progressive Web Apps (PWA)
- Serverless architectures enable dynamic content delivery without managing servers directly, ideal for scalable frontend systems like multi-tenant platforms (e.g., Miro boards).
- PWAs combine web technologies with native app capabilities, offering offline access and native-like performance.
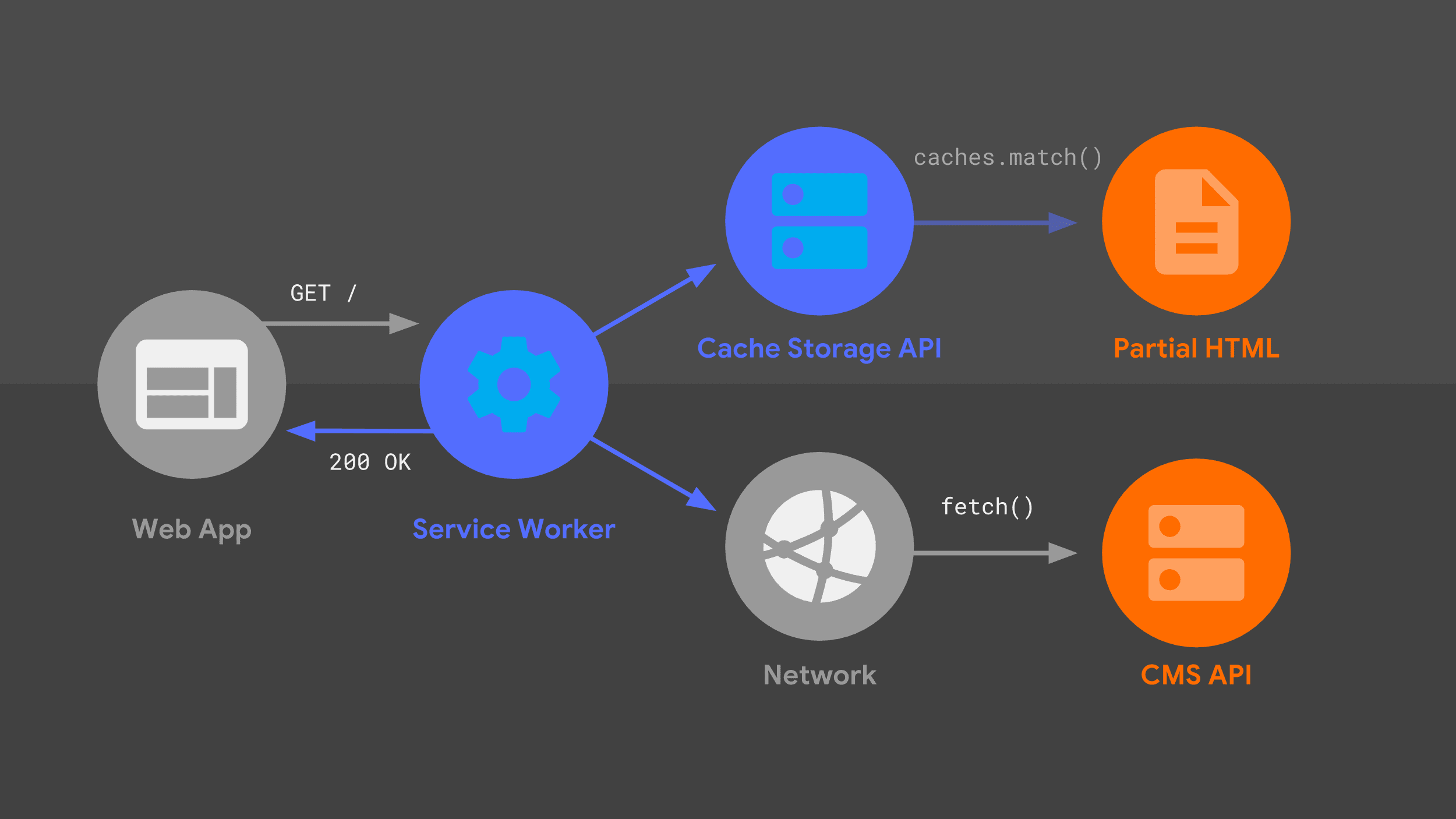
State Management
State management is essential in frontend apps to track UI data changes, especially in SPAs. Popular patterns include:
- Flux/Redux: Centralized state management using unidirectional data flow.
- Context API: React’s built-in mechanism to share state between components without prop-drilling.
Quote: “Choosing the right state management approach is critical to a scalable frontend architecture. Redux provides predictability but can increase complexity.” — Frontend Lead, Tech Journal
Security, Cookies, and Authentication
Security practices include managing cookies and tokens correctly to prevent vulnerabilities:
- Use HTTP-Only Cookies to protect sensitive session information.
- Implement OAuth2.0 and JWT for secure authentication.
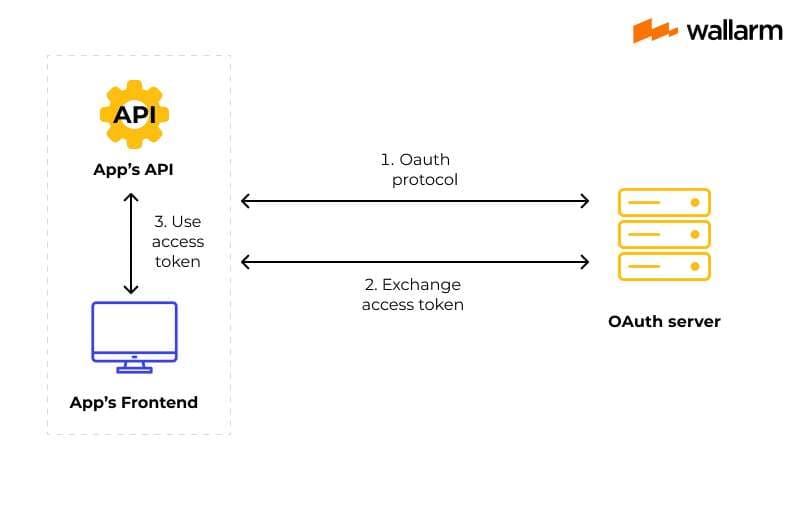
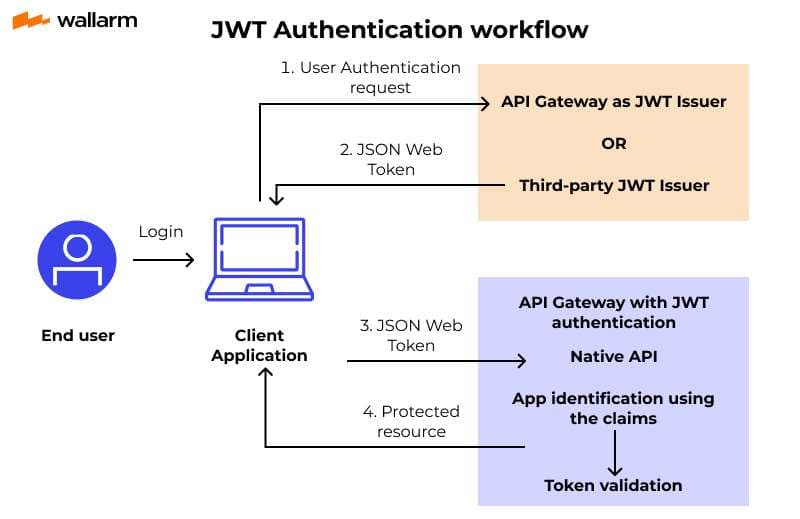
Principles:
- Adopt CORS policies to secure frontend-backend interactions.
- Ensure CSRF protection in multi-tenant platforms.
Backend Architecture Conventions and Principles
Backend architecture focuses on managing data, handling business logic, and ensuring secure, scalable, and reliable operations. Let's explore the guiding principles:
Authentication (AuthN) and Authorization (AuthZ)
Implementing secure authentication and authorization is critical in modern systems:
- Use OAuth2 for delegation.
- Implement RBAC (Role-Based Access Control) to control permissions.
Authentication and Authorization
Principle: Authentication and authorization should be handled separately to avoid security risks. Employ industry standards like JWT or SAML.
Scaling and Distributed Services
Modern backend architectures are designed for scalability, especially for applications like social media or eCommerce platforms:
- Horizontal scaling involves adding more servers to distribute the load.
- Implement distributed services using microservices patterns.
Scaling Distributed Services in Backend
Principles:
- Use load balancers and auto-scaling mechanisms to dynamically adjust to traffic.
- Adopt sharding for database scaling in high-transaction systems.
Data Consistency, Validity, and Availability
In distributed systems, achieving data consistency and availability is a challenge, particularly in systems like banking and healthcare.
- Employ the CAP theorem to manage trade-offs between Consistency, Availability, and Partition Tolerance.
- Use ACID properties for data consistency in critical systems and BASE principles for eventual consistency in high-availability systems.
Principle: Prioritize data consistency for systems where the accuracy of the data is non-negotiable, such as financial applications.
Data Consistency and Availability
Security and Data Protection
Security remains a top priority across all backend systems:
- Use encryption (SSL/TLS) for data in transit.
- Implement encryption-at-rest for sensitive data, especially in industries like finance.
Principle: Regular security audits and adopting DevSecOps practices can greatly enhance system security.
Conclusion
In this post, we explored fundamental conventions and principles in frontend and backend architectures, focusing on scalability, state management, security, and data integrity. These principles ensure your systems remain robust, maintainable, and scalable as your application evolves.
In the next part, we’ll delve into Solution Architecture, providing a deeper understanding of how individual system components come together to solve complex problems.
Appendix: Common Architecture Conventions and Principles
Convention/Principle | Description | Example | Area |
---|---|---|---|
Single Responsibility | Each module/class should have one and only one reason to change | Separation of business logic | Backend |
Separation of Concerns | Divide an application so that each part addresses a specific concern | UI vs business logic vs data access | Frontend |
DRY (Don’t Repeat Yourself) | Reduce repetition of code patterns by modularizing functionalities | Utility functions for repetitive tasks | Frontend/Backend |
KISS (Keep It Simple) | Keep the design simple and avoid unnecessary complexity | Flat component structure in frontend | Frontend |
Statelessness | Ensure APIs don’t maintain any session state | RESTful APIs | Backend |
ACID Properties | Guarantees for database transactions ensuring atomicity, consistency, isolation, durability | Transaction management in banking apps | Backend |
CAP Theorem | In distributed systems, you can only achieve two out of consistency, availability, and partition tolerance | Databases for large-scale systems | Backend |
Responsive Design | Design web applications to be accessible across different device sizes | Mobile-first design | Frontend |